Rest API Testing Using Postman
Postman is a very good tool for testing rest APIs. It can be used backend as well as frontend developer for Rest APIs testing. We can do Mannual as well as Automation testing using Postman.
Below is the URL for download the postman https://www.postman.com/downloads
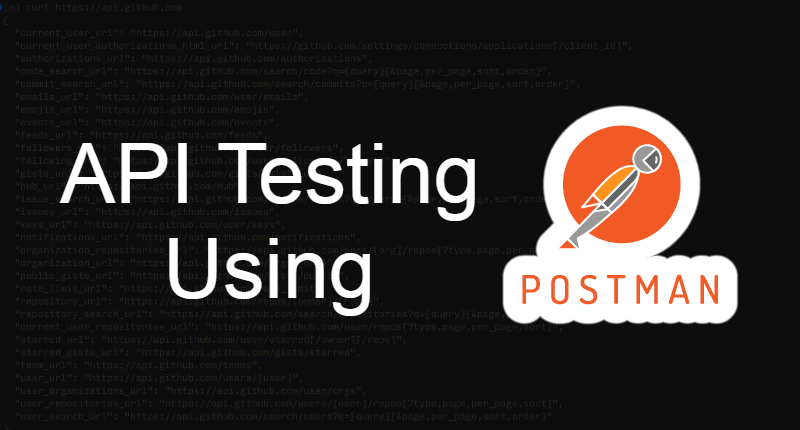
For the Rest API Testing we use below url https://reqres.in
Once we setup the postman lets start implementing Unit test cases.
Video Demo is present on https://youtu.be/_NkOiv-aid8
List User API
Request URL:- https://reqres.in/api/users
Method:- GET
Request:-
Response Body:-
{ "page": 1, "per_page": 6, "total": 12, "total_pages": 2, "data": [ { "id": 1, "email": "george.bluth@reqres.in", "first_name": "George", "last_name": "Bluth", "avatar": "https://reqres.in/img/faces/1-image.jpg" }, { "id": 2, "email": "janet.weaver@reqres.in", "first_name": "Janet", "last_name": "Weaver", "avatar": "https://reqres.in/img/faces/2-image.jpg" }, { "id": 3, "email": "emma.wong@reqres.in", "first_name": "Emma", "last_name": "Wong", "avatar": "https://reqres.in/img/faces/3-image.jpg" }, { "id": 4, "email": "eve.holt@reqres.in", "first_name": "Eve", "last_name": "Holt", "avatar": "https://reqres.in/img/faces/4-image.jpg" }, { "id": 5, "email": "charles.morris@reqres.in", "first_name": "Charles", "last_name": "Morris", "avatar": "https://reqres.in/img/faces/5-image.jpg" }, { "id": 6, "email": "tracey.ramos@reqres.in", "first_name": "Tracey", "last_name": "Ramos", "avatar": "https://reqres.in/img/faces/6-image.jpg" } ] }
Unit Test Cases:-
const response = JSON.parse(responseBody); pm.test("Status code is 200", function () { pm.response.to.have.status(200); }); pm.test("Should have Data", () => { pm.expect(response.data.length).is.gt(0); });
Unit Test Cases Result:-
Get Single User (Get Data for ID 2)
Request URL:- https://reqres.in/api/users/2
Method:- GET
Response Body:-
{ "data": { "id": 2, "email": "janet.weaver@reqres.in", "first_name": "Janet", "last_name": "Weaver", "avatar": "https://reqres.in/img/faces/2-image.jpg" } }
Unit Test Cases:-
>const response = JSON.parse(responseBody); pm.test("Status code is 200", function () { pm.response.to.have.status(200); }); pm.test("Should have Id 2", () => { pm.expect(response.data.id).is.eq(2); });
Unit Test Cases Result:-
Create Single User
Request URL:- https://reqres.in/api/users
Method:- POST
Request Body:-
{ "name": "morpheus", "job": "leader" }
Response Body:-
{ "name": "morpheus", "job": "leader", "id": "319", "createdAt": "2022-04-30T18:45:45.080Z" }
Unit Test Cases:-
const response = JSON.parse(responseBody); pm.test("Status code is 201", function () { pm.response.to.have.status(201); }); pm.test("Should not have blank response", () => { pm.expect(typeof response.name).is.not.eq(undefined); pm.expect(typeof response.job).is.not.eq(undefined); pm.expect(typeof response.id).is.not.eq(undefined); pm.expect(typeof response.createdAt).is.not.eq(undefined); });
Unit Test Cases Results:-
Update Single User
Request URL:- https://reqres.in/api/users
Method:- PUT
Request Body:-
{ "name": "morpheus", "job": "leader" }
Response Body:-
{ "name": "morpheus", "job": "leader", "updatedAt": "2022-04-30T18:46:35.881Z" }
Unit Test Cases:-
const response = JSON.parse(responseBody); pm.test("Status code is 200", function () { pm.response.to.have.status(200); }); pm.test("Should not have blank response", () => { pm.expect(typeof response.name).is.not.eq(undefined); pm.expect(typeof response.job).is.not.eq(undefined); pm.expect(typeof response.updatedAt).is.not.eq(undefined); });
Unit Test Cases Results:-
Delete Single User
Request URL:- https://reqres.in/api/users/2
Method:- DELETE
Unit Test Cases:-
pm.test("Status code is 204", function () { pm.response.to.have.status(204); });
Unit Test Cases Results:-
{ "info": { "_postman_id": "794515f9-2e14-434c-a50b-20c677e84da4", "name": "Test Postman Collection", "schema": "https://schema.getpostman.com/json/collection/v2.1.0/collection.json" }, "item": [{ "name": "List Users", "event": [{ "listen": "test", "script": { "exec": [ "const response = JSON.parse(responseBody);", "", "pm.test(\"Status code is 200\", function () {", " pm.response.to.have.status(200);", "});", "", "pm.test(\"Should have Data\", () => {", " pm.expect(response.data.length).is.gt(0);", "});" ], "type": "text/javascript" } }], "request": { "method": "GET", "header": [], "url": { "raw": "https://reqres.in/api/users", "protocol": "https", "host": [ "reqres", "in" ], "path": [ "api", "users" ] } }, "response": [] }, { "name": "Get Single User", "event": [{ "listen": "test", "script": { "exec": [ "const response = JSON.parse(responseBody);", "", "pm.test(\"Status code is 200\", function () {", " pm.response.to.have.status(200);", "});", "", "pm.test(\"Should have Id 2\", () => {", " pm.expect(response.data.id).is.eq(2);", "});" ], "type": "text/javascript" } }], "request": { "method": "GET", "header": [], "url": { "raw": "https://reqres.in/api/users/2", "protocol": "https", "host": [ "reqres", "in" ], "path": [ "api", "users", "2" ] } }, "response": [] }, { "name": "Get Single User Not Found", "event": [{ "listen": "test", "script": { "exec": [ "const response = JSON.parse(responseBody);", "", "pm.test(\"Status code is 404\", function () {", " pm.response.to.have.status(404);", "});", "", "pm.test(\"Should have blank\", () => {", " pm.expect(Object.keys(response).length).is.eq(0);", "});" ], "type": "text/javascript" } }], "request": { "method": "GET", "header": [], "url": { "raw": "https://reqres.in/api/users/23", "protocol": "https", "host": [ "reqres", "in" ], "path": [ "api", "users", "23" ] } }, "response": [] }, { "name": "Create Single User", "event": [{ "listen": "test", "script": { "exec": [ "const response = JSON.parse(responseBody);", "", "pm.test(\"Status code is 201\", function () {", " pm.response.to.have.status(201);", "});", "", "pm.test(\"Should not have blank response\", () => {", " pm.expect(typeof response.name).is.not.eq(undefined);", " pm.expect(typeof response.job).is.not.eq(undefined);", " pm.expect(typeof response.id).is.not.eq(undefined);", " pm.expect(typeof response.createdAt).is.not.eq(undefined);", "});" ], "type": "text/javascript" } }], "request": { "method": "POST", "header": [], "body": { "mode": "raw", "raw": "{\n \"name\": \"morpheus\",\n \"job\": \"leader\"\n}", "options": { "raw": { "language": "json" } } }, "url": { "raw": "https://reqres.in/api/users", "protocol": "https", "host": [ "reqres", "in" ], "path": [ "api", "users" ] } }, "response": [] }, { "name": "Update Single User", "event": [{ "listen": "test", "script": { "exec": [ "const response = JSON.parse(responseBody);", "", "pm.test(\"Status code is 200\", function () {", " pm.response.to.have.status(200);", "});", "", "pm.test(\"Should not have blank response\", () => {", " pm.expect(typeof response.name).is.not.eq(undefined);", " pm.expect(typeof response.job).is.not.eq(undefined);", " pm.expect(typeof response.updatedAt).is.not.eq(undefined);", "});" ], "type": "text/javascript" } }], "request": { "method": "PUT", "header": [], "body": { "mode": "raw", "raw": "{\n \"name\": \"morpheus\",\n \"job\": \"leader\"\n}", "options": { "raw": { "language": "json" } } }, "url": { "raw": "https://reqres.in/api/users", "protocol": "https", "host": [ "reqres", "in" ], "path": [ "api", "users" ] } }, "response": [] }, { "name": "Delete Single User", "event": [{ "listen": "test", "script": { "exec": [ "pm.test(\"Status code is 204\", function () {", " pm.response.to.have.status(204);", "});", "" ], "type": "text/javascript" } }], "request": { "method": "DELETE", "header": [], "url": { "raw": "https://reqres.in/api/users/2", "protocol": "https", "host": [ "reqres", "in" ], "path": [ "api", "users", "2" ] } }, "response": [] } ] }
Comments
Post a Comment